08-23-2018 03:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
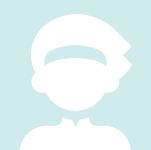
08-23-2018 03:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
import * as messaging from "messaging";
// Check peerSocket values
console.log("app messaging.peerSocket.readyState: "+ messaging.peerSocket.readyState);
console.log("app messaging.peerSocket.OPEN: "+ messaging.peerSocket.OPEN);
// Request weather data from the companion
function fetchWeather() {
if (messaging.peerSocket.readyState === messaging.peerSocket.OPEN) {
// Send a command to the companion
messaging.peerSocket.send({
command: 'weather'
});
}
}
// Listen for the onopen event
messaging.peerSocket.onopen = function() {
// Fetch weather when the connection opens
fetchWeather();
}
// Listen for messages from the companion
messaging.peerSocket.onmessage = function(evt) {
if (evt.data) {
console.log("Data found: "+evt.data);
}
}
// Listen for the onerror event
messaging.peerSocket.onerror = function(err) {
// Handle any errors
console.log("Connection error: " + err.code + " - " + err.message);
}
// Fetch the weather every 30 minutes
setInterval(fetchWeather, 30 * 1000 * 60);
import * as messaging from "messaging";
// Send the weather data to the device
function returnWeatherData(data) {
if (messaging.peerSocket.readyState === messaging.peerSocket.OPEN) {
// Send a command to the device
messaging.peerSocket.send("hello from companion");
} else {
console.log("Error: Connection is not open");
}
}
// Listen for messages from the device
messaging.peerSocket.onmessage = function(evt) {
if (evt.data && evt.data.command == "weather") {
// The device requested weather data
console.log("The device requested weather data");
}
}
// Listen for the onerror event
messaging.peerSocket.onerror = function(err) {
// Handle any errors
console.log("Connection error: " + err.code + " - " + err.message);
}
09-01-2018 10:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

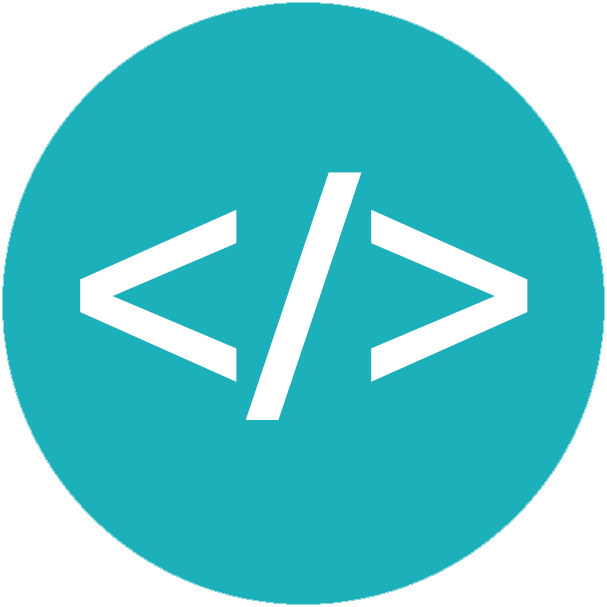
09-01-2018 10:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Here's a minimal example, check it's working for you:
device/index.js
import * as messaging from "messaging"; messaging.peerSocket.onopen = function() { console.log(`Device OPEN`); sendMessage(); } messaging.peerSocket.onerror = function(err) { console.log(`Device ERROR: ${err.code} ${err.message}`); } function sendMessage() { console.log(`Device SEND`); if (messaging.peerSocket.readyState === messaging.peerSocket.OPEN) { messaging.peerSocket.send({cmd: "ping!"}); } else { console.log("Dude, where's my peerSocket?"); } }
companion/index.js
import * as messaging from "messaging"; messaging.peerSocket.onopen = function() { console.log(`Companion OPEN`); } messaging.peerSocket.onerror = function(err) { console.log(`Companion ERROR: ${err.code} ${err.message}`); } messaging.peerSocket.onmessage = function(evt) { console.log(`Companion MESSAGE: ${JSON.stringify(evt.data)}`); }

08-12-2020 08:28
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
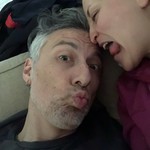
08-12-2020 08:28
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Actually I have the same problem.
When connecting to the simulator, your minimal example works.
However, using my Versa 2, it no longer works. The onopen isn't even called.
08-13-2020 06:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
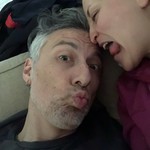
08-13-2020 06:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Remark to my own post: I solved the issue. 'Solved', since I removed the dev apps, restarted my iPhone and reinstalled the app again. Then it works.
Something to look at for the fitibit devs! 😉

