05-13-2018 16:33
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-13-2018 16:33
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
So far I have imported my steps into the watch face, but I notice that it only updates on my watch face when I swipe up to view the steps that the fitbit shows. How do I make this continually update?
let stepsLabel = document.getElementById("steps");
console.log((today.local.steps || 0) + " Steps");
stepsLabel.text = ((today.local.steps || 0) + " Steps");
Answered! Go to the Best Answer.

Accepted Solutions
05-16-2018 21:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-16-2018 21:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Thanks for your help. I actually just renamed today to "thisday" for the time portion of that code and that seemed to work.
let thisday = evt.date; let hours = thisday.getHours(); let sec = thisday.getSeconds();
and then used today for my steps, distance etc.
let stepsLabel = document.getElementById("stepsLabel"); console.log((today.local.steps || 0) + " Steps"); stepsLabel.text = ((today.local.steps || 0) + " Steps");
05-13-2018 17:26
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-13-2018 17:26
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Your code needs to keep updating by either calling through the on the clock tick probably a function called updateClock in this example:
clock.ontick = () => updateClock();
Or in a function that is called on an interval; like updateClockData in this example:
setInterval(updateClockData, .5*1000);
The advantage here is that my updateClock is only called once per minute, but updateClockData is called twice a second (which might be a bit aggressive; but I wanted a widget to move somewhat quickly on this face; namely a needle that shows your relative heart rate).

05-13-2018 17:30
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-13-2018 17:30
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Also if you use
today.adjusted.steps
instead of
today.local.steps
You will get the fitbit account value instead of just the value from your watch. This can fall out of sync if you have multiple devices.

05-13-2018 20:59
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-13-2018 20:59
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Thanks I created a separate function that now updates my steps every second, but this function is outside of my clock function, and that seems to have stopped my watch from reading time, do they need to be inside the same function?
clock.ontick = (evt) => { let today = evt.date; let hours = today.getHours(); let sec = today.getSeconds(); let year = today.getYear(); let monthnum = today.getMonth(); let day = today.getDate(); var month = new Array(); month[0] = "01"; month[1] = "02"; month[2] = "03"; month[3] = "04"; month[4] = "05"; month[5] = "06"; month[6] = "07"; month[7] = "08"; month[8] = "09"; month[9] = "10"; month[10] = "11"; month[11] = "12"; let monthname = month[monthnum]; if (preferences.clockDisplay === "12h") { // 12h format hours = hours % 12 || 12; } else { // 24h format hours = util.zeroPad(hours); } let mins = util.zeroPad(today.getMinutes()); let sec = util.zeroPad(today.getSeconds()); //myLabel.text = (hours ":" (today.getMinutes()) ":" (today.getSeconds()) ); myLabel.text = `${hours}:${mins}:${sec}`; myMonth.text = `${monthname}-${day}-${year}`; myDay.text = `${day}`; //myYear.text = `${year}`; };
clock.ontick = function(updateSteps){ //steps let stepsLabel = document.getElementById("steps"); console.log((today.local.steps || 0) + " Steps"); stepsLabel.text = ((today.local.steps || 0) + " Steps"); //floors let stairsLabel = document.getElementById("stairs"); console.log(today.local.eleviationGain || 0); stairsLabel.text = ((today.local.elevationGain || 0) + " Floors"); }

05-14-2018 04:38
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-14-2018 04:38
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
You can only have 1 on onTick function. you can put the code from update steps directly into the updateClock or have the last step of updateClock be a call to updateSteps.
A clean/isolated approach I might suggest to one of my students would be something like this:
clock.ontick = () => doUpdates();
Then doUpdates:
function doUpdates(){ updateClock(); updateStats() }
Next updateClock:
upDateClock(){
let today = new Date();
let hours = today.getHours(); let sec = today.getSeconds(); let year = today.getYear(); let month = util.zeroPad(today.getMonth()); let day = today.getDate(); if (preferences.clockDisplay === "12h") { // 12h format hours = hours % 12 || 12; } else { // 24h format hours = util.zeroPad(hours); } let mins = util.zeroPad(today.getMinutes()); let sec = util.zeroPad(today.getSeconds()); //myLabel.text = (hours ":" (today.getMinutes()) ":" (today.getSeconds()) ); myLabel.text = `${hours}:${mins}:${sec}`; myMonth.text = `${monthname}-${day}-${year}`; myDay.text = `${day}`; //myYear.text = `${year}`; };
finally updateStats:
function updateStats(){
//steps
let stepsLabel = document.getElementById("steps"); console.log((today.local.steps || 0) + " Steps"); stepsLabel.text = ((today.local.steps || 0) + " Steps");
//floors let stairsLabel = document.getElementById("stairs"); console.log(today.local.eleviationGain || 0); stairsLabel.text = ((today.local.elevationGain || 0) + " Floors");
}

05-14-2018 19:36
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-14-2018 19:36
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Thanks, I'm still having issues with nothing updating with this method.
clock.ontick = () => doUpdates(); function doUpdates(){ updateClock(); updateStats(); } // Clock function updateClock(){ clock.granularity = "seconds"; let today = evt.date; let hours = today.getHours(); let sec = today.getSeconds(); let year = today.getYear()-100; let monthnum = today.getMonth(); let day = today.getDate(); let weeknum = today; var weekday = new Array(); weekday[0] = "Sun"; weekday[1] = "Mon"; weekday[2] = "Tues"; weekday[3] = "Wed"; weekday[4] = "Thur"; weekday[5] = "Fri"; weekday[6] = "Sat"; let weekname = weekday[weeknum]; var month = new Array(); month[0] = "01"; month[1] = "02"; month[2] = "03"; month[3] = "04"; month[4] = "05"; month[5] = "06"; month[6] = "07"; month[7] = "08"; month[8] = "09"; month[9] = "10"; month[10] = "11"; month[11] = "12"; let monthname = month[monthnum]; if (preferences.clockDisplay === "12h") { // 12h format hours = hours % 12 || 12; } else { // 24h format hours = util.zeroPad(hours); } let mins = util.zeroPad(today.getMinutes()); let sec = util.zeroPad(today.getSeconds()); myLabel.text = `${hours}:${mins}:${sec}`; myMonth.text = `${monthname}-${day}-${year}`; myDay.text = `${weekname}`; }
Then I have this.
function updateStats(){ //steps let mySteps = document.getElementById("mySteps"); console.log((today.local.steps || 0) + " Steps"); mySteps.text = ((today.local.steps || 0) + " Steps"); //floors let myStairs = document.getElementById("myStairs"); console.log(today.local.eleviationGain || 0); myStairs.text = ((today.local.elevationGain || 0) + " Floors"); // Battery let batteryLabel = document.getElementById("battery"); console.log(Math.floor(battery.chargeLevel) + "%"); //retreive battery data batteryLabel.text = ((battery.chargeLevel) + "%"); // send battery data to index.gui };
When I run the app nothing is updating on the console log...

05-14-2018 19:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-14-2018 19:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Not on my computer right now, but I want to say it is that the line:
clock.granularity = "seconds";
Needs to be outside of any function. Otherwise it only gets set after the fun3is called... And it is never called since it is never set.

05-14-2018 21:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-14-2018 21:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I moved that line outside the function. Now it is telling me that "evt" is not defined.
I believe that this has something to do with the following line
let today = evt.date;
Since the onclick function is now referring to
clock.ontick = () => doUpdates();
and "evt" is no longer initialized.

05-15-2018 02:48 - edited 05-15-2018 02:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-15-2018 02:48 - edited 05-15-2018 02:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
To fix that I would change
let today = evt.date;
To
let today = new Date();

05-15-2018 04:01
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
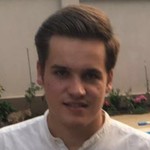
05-15-2018 04:01
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
clock.granularity = "seconds";
clock.ontick = (evt) => {
let today = evt.date; let hours = today.getHours(); let sec = today.getSeconds(); let year = today.getYear(); let monthnum = today.getMonth(); let day = today.getDate(); var month = new Array(); month[0] = "01"; month[1] = "02"; month[2] = "03"; month[3] = "04"; month[4] = "05"; month[5] = "06"; month[6] = "07"; month[7] = "08"; month[8] = "09"; month[9] = "10"; month[10] = "11"; month[11] = "12"; let monthname = month[monthnum]; if (preferences.clockDisplay === "12h") { // 12h format hours = hours % 12 || 12; } else { // 24h format hours = util.zeroPad(hours); } let mins = util.zeroPad(today.getMinutes()); let sec = util.zeroPad(today.getSeconds()); //myLabel.text = (hours ":" (today.getMinutes()) ":" (today.getSeconds()) ); myLabel.text = `${hours}:${mins}:${sec}`; myMonth.text = `${monthname}-${day}-${year}`; myDay.text = `${day}`;
let stepsLabel = document.getElementById("steps"); stepsLabel.text = ((today.local.steps || 0) + " Steps"); let stairsLabel = document.getElementById("stairs"); stairsLabel.text = ((today.local.elevationGain || 0) + " Floors");
};
Try it like this, you can use ontick only once, do everything in one ontick, update the date, the time and the stats 🙂

05-16-2018 21:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

05-16-2018 21:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Thanks for your help. I actually just renamed today to "thisday" for the time portion of that code and that seemed to work.
let thisday = evt.date; let hours = thisday.getHours(); let sec = thisday.getSeconds();
and then used today for my steps, distance etc.
let stepsLabel = document.getElementById("stepsLabel"); console.log((today.local.steps || 0) + " Steps"); stepsLabel.text = ((today.local.steps || 0) + " Steps");
05-29-2019 17:44
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
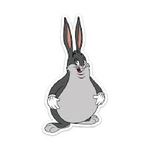
05-29-2019 17:44
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
what is the variable name for the steps

05-31-2019 05:34 - edited 05-31-2019 05:36
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

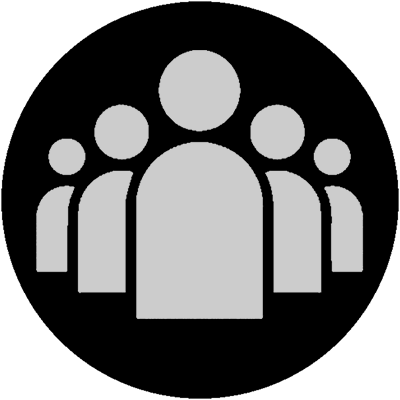
05-31-2019 05:34 - edited 05-31-2019 05:36
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Reference is my Really Basic clockface
import userActivity from "user-activity"; //adjusted types (matching the stats that you upload to fitbit.com, as opposed to local types) let stepsValue = (userActivity.today.adjusted["steps"] || 0); // steps value measured from fitbit is assigned to the variable stepsValue console.log("steps: " + stepsValue);

09-22-2020 17:17
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
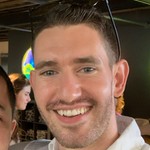
09-22-2020 17:17
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Thank you, Dcable! I couldn't get this to work any other way, either!
I was able to keep it a bit simpler with the line:
mySteps.text = today.adjusted.steps;
But the "today" wasn't working until I changed it to:
let thisday = evt.date;
For some reason you couldn't reuse the "today" in both instances.
New code for me:
clock.ontick = (evt) => {
let thisday = evt.date;
let hours = thisday.getHours();
let hours = hours % 12 || 12;
let mins = zeroPad(thisday.getMinutes());
myLabel.text = `${hours}:${mins}`;
myBat.text = battery.chargeLevel + "%";
//convert date to string and slice from left start 0 to 10 characters
myDate.text = thisday.toString().slice(0, 10);
heartSensor.start();
mySteps.text = today.adjusted.steps;
