03-28-2019 02:31
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
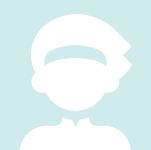
03-28-2019 02:31
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Hey everyone,
for an application I need a more or less continuous stream of accelerometer data. I want the app to run all the time so I use a clockface. My approach is to use an accelerometer with 20 Hz and a batch size of 20 (so every second there's a readout).
Whenever the accelerometer has a readout, I message it to the Companion.
My problem is that the messaging seems to be very slow. So when the Companion received the first package, 5 more were sent. This leads to the unpleasant situation that I send 5 times more data than I receive. When the clockface crashes, all unreceived packages are lost.
I am really out of my wits and very grateful for any ideas.
Best
Simon

03-28-2019 11:50
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

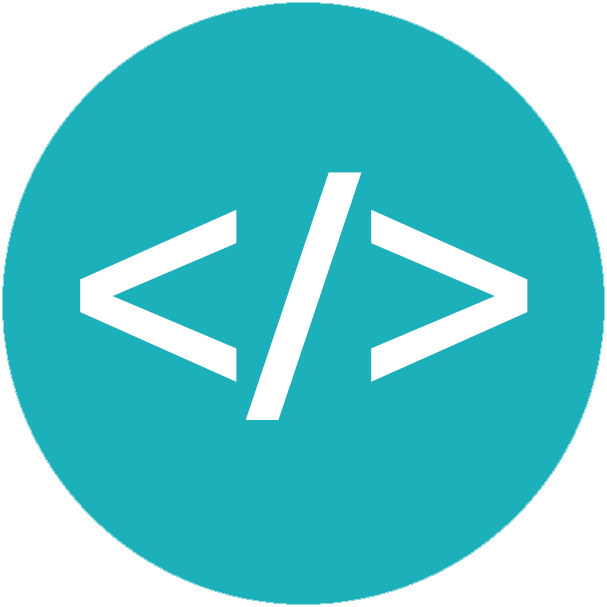
03-28-2019 11:50
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Can you provide some code for your project? What format data are you sending?
It might be worth using an approach like this: https://www.npmjs.com/package/fitbit-asap

04-01-2019 01:38 - edited 04-01-2019 01:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
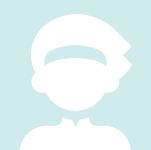
04-01-2019 01:38 - edited 04-01-2019 01:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Hey Jon,
sorry for the late reply. Got stuck in something... . I changed my sending strategy such that now I have a send/received ratio of 2/1 when I am constantly sending data from device to Companion. I am sending JSONs which I later forward to a web API.
I attach some code and thank you very much in advance for input.
Btw. the asap package is awesome, thanks for sharing it.
Cheers
Simon
#### APP ####
import asap from "fitbit-asap/app" import { me } from "appbit"; import { Accelerometer } from "accelerometer"; import { Gyroscope } from "gyroscope"; // Disable app timeout if (me.appTimeoutEnabled) { console.log("Timeout is enabled. Disabling it!"); me.appTimeoutEnabled = false; // Disable timeout } else { console.log("Timeout is disabled. Nice!") } // sensor settings const samplerate = 20; const batch = 20; const settings = { frequency: samplerate, batch: batch }; // initialize sensors let acc = new Accelerometer(settings); let gyro = new Gyroscope(settings); // norm function for sensors function getNorm(x, y, z) { return Math.sqrt(Math.pow(x, 2) + Math.pow(y, 2) + Math.pow(z, 2)) } // aux sender function function send(data){ // asap is more stable messaging // https://www.npmjs.com/package/fitbit-asap asap.send(data) }
// will later be sent to Companion var send_array = {}
// serves as message ID var counter = 0 acc.addEventListener("reading", () => { // initialize sending objects var data = {}; data['endtime'] = new Date().getTime(); for (let index = 0; index < acc.readings.timestamp.length; index++) { data[acc.readings.timestamp[index]] = getNorm(acc.readings.x[index], acc.readings.y[index], acc.readings.z[index]) }; send_array['a'] = data } ) gyro.addEventListener("reading", () => { // initialize sending objects var data = {}; data['endtime'] = new Date().getTime(); for (let index = 0; index < gyro.readings.timestamp.length; index++) { data[gyro.readings.timestamp[index]] = getNorm(gyro.readings.x[index], gyro.readings.y[index], gyro.readings.z[index]) }; send_array['g'] = data send_array['ID'] = counter.toString() send(send_array) counter = counter + 1 send_array = {} } ) acc.start() gyro.start()
#### COMPANION ####
import asap from "fitbit-asap/companion" import * as messaging from "messaging"; import { me as companion } from "companion"; // to upload var uploadArray = [] // append incoming data to postData asap.onmessage = function(evt) { const message = evt.data; // print chunk ID console.log('Companion: Receiving ' + message['ID']); uploadArray.push(message) if (uploadArray.length == 30 ){ console.log('Got ' + uploadArray.length.toString() + ' seconds') // upload to API const formData = new FormData(); formData.append('name', 'New Approach ' + message['ID']) formData.append('json_string', uploadArray) fetch('https://my_api_adress', {method: 'POST', body: formData}) uploadArray = [] } }

