10-06-2019 06:08
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
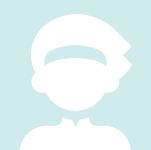
10-06-2019 06:08
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- ? at app/index.js:27,7
// Get a handle on the <text> elements specified in the index.gui file
const timeHandle = document.getElementById("timeLabel");
const stepsHandle = document.getElementById("stepsLabel");
const dayHandle = document.getElementById ("dayLabel");
const monthHandle = document.getElementById ("monthLabel");
const daynameHandle = documnet.getElementById ("daynameLabel");
// Update the <text> element every tick with the current time
clock.ontick = (evt) => {
let today = new Date();
let monthnum = today.getMonth();
let day = today.getDate();
var month = new Array();
month[0] = "Jan";
month[1] = "Feb";
month[2] = "Mar";
month[3] = "Apr";
month[4] = "May";
month[5] = "Jun";
month[6] = "Jul";
month[7] = "Aug";
month[8] = "Sep";
month[9] = "Oct";
month[10] = "Nov";
month[11] = "Dec";
let monthname = month[monthnum];
var day = new Array();
day [0] = "Mon";
day [1] = "Tue";
day [2] = "Wed";
day [3] = "Thu";
day [4] = "Fri";
day [5] = "Sat";
day [6] = "Sun";
let dayname = day[today.getDate];
const now = evt.date;
let hours = now.getHours();
let mins = now.getMinutes();
if (preferences.clockDisplay === "12h") {
hours = hours % 12 || 12;
} else {
hours = zeroPad(hours);
}
let minsZeroed = zeroPad(mins);
timeHandle.text = `${hours}:${minsZeroed}`;
monthHandle.text = `${monthname}`;
dayHandle.text = `${day}`;
daynameHandle.text = `${dayname}`;
// Activity Values: adjusted type
let stepsValue = (userActivity.today.adjusted["steps"] || 0);
let stepsString = stepsValue + ' steps';
stepsHandle.text = stepsString;
}
/* -------- SETTINGS -------- */
let stepsLabel = document.getElementById("stepsLabel");
let timeLabel = document.getElementById("timeLabel");
let dayLabel = document.getElementById("dayLabel");
let monthLabel = document.getElementById("monthLabel");
let dividerTop = document.getElementById ("divider-top");
let dividerBtm = document.getElementById ("divider-btm");
let daynameLabel = dcument.getElementById ("daynameLabel");
function settingsCallback(data){
if (!data){
return;
}
if (data.colorSteps) {
stepsLabel.style.fill = data.colorSteps;
}
if (data.colorTime) {
timeLabel.style.fill = data.colorTime;
}
if (data.colorDayname) {
daynameLabel.style.fill = data.colorDayname;
}
if (data.colorDay) {
dayLabel.style.fill = data.colorDay;
}
if (data.colorMonth) {
monthLabel.style.fill = data.colorMonth;
}
if (data.colorDivider) {
dividerTop.style.fill = data.colorDivider;
dividerBtm.style.fill = data.colorDivider;
}
}
simpleSettings.initialize(settingsCallback);

10-06-2019 09:35
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
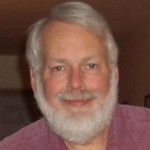
10-06-2019 09:35
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
You appear to have at least 2 typos in your code:
1) The message that documnet is not defined says that you misspelled document somewhere, but I don't see it it in this code, and
2) You have a line that says "
let daynameLabel = dcument.getElementById ("daynameLabel");
I think you meant to type document instead of dcument.
Please review your code carefully for spelling errors.
John

10-06-2019 11:13
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
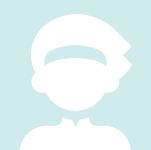
10-06-2019 11:13
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
const daynameHandle = documnet.getElementById ("daynameLabel");
And typos in other places as well...
Since document.getElementById name is long and prone to typos, just use a shorter delegate.
In my case:
function $(id) {
return document.getElementById(id)
}
// Usage:
const myElement = $('myElementId')

10-07-2019 02:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
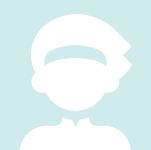
10-07-2019 02:43
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I am having a few issues with getting the weekday to display.
Instead of display Mon, Tue, Wed, Thu, Fri, Sat or Sun it is displaying underlined.
const timeHandle = document.getElementById("timeLabel");
const stepsHandle = document.getElementById("stepsLabel");
const dayHandle = document.getElementById ("dayLabel");
const monthHandle = document.getElementById ("monthLabel");
const daynameHandle = document.getElementById ("daynameLabel");
// Update the <text> element every tick with the current time
clock.ontick = (evt) => {
let today = new Date();
let monthnum = today.getMonth();
let dayname = today.getDay();
let day = today.getDate();
var month = new Array();
month[0] = "Jan";
month[1] = "Feb";
month[2] = "Mar";
month[3] = "Apr";
month[4] = "May";
month[5] = "Jun";
month[6] = "Jul";
month[7] = "Aug";
month[8] = "Sep";
month[9] = "Oct";
month[10] = "Nov";
month[11] = "Dec";
let monthname = month[monthnum];
var dayname = new Array();
day[0] = "Mon";
day[1] = "Tue";
day[2] = "Wed";
day[3] = "Thu";
day[4] = "Fri";
day[5] = "Sat";
day[6] = "Sun";
let dayname = day[today.getDay]
const now = evt.date;
let hours = now.getHours();
let mins = now.getMinutes();
if (preferences.clockDisplay === "12h") {
hours = hours % 12 || 12;
} else {
hours = zeroPad(hours);
}
let minsZeroed = zeroPad(mins);
timeHandle.text = `${hours}:${minsZeroed}`;
monthHandle.text = `${monthname}`;
dayHandle.text = `${day}`;
daynameHandle.text = `${dayname}`;
// Activity Values: adjusted type
let stepsValue = (userActivity.today.adjusted["steps"] || 0);
let stepsString = stepsValue + ' steps';
stepsHandle.text = stepsString;
}
/* -------- SETTINGS -------- */
let stepsLabel = document.getElementById("stepsLabel");
let timeLabel = document.getElementById("timeLabel");
let dayLabel = document.getElementById("dayLabel");
let monthLabel = document.getElementById("monthLabel");
let dividerTop = document.getElementById ("divider-top");
let dividerBtm = document.getElementById ("divider-btm");
let daynameLabel = document.getElementById ("daynameLabel");
function settingsCallback(data){
if (!data){
return;
}
if (data.colorSteps) {
stepsLabel.style.fill = data.colorSteps;
}
if (data.colorTime) {
timeLabel.style.fill = data.colorTime;
}
if (data.colorDayname) {
daynameLabel.style.fill = data.colorDayname;
}
if (data.colorDay) {
dayLabel.style.fill = data.colorDay;
}
if (data.colorMonth) {
monthLabel.style.fill = data.colorMonth;
}
if (data.colorDivider) {
dividerTop.style.fill = data.colorDivider;
dividerBtm.style.fill = data.colorDivider;
}
}
simpleSettings.initialize(settingsCallback);
I am still receiving these two errors but I can't find the type errors
TypeError: Cannot read property 'Symbol(Symbol.iterator)' of undefined
Uncaught TypeError: Cannot read property 'Symbol(Symbol.iterator)' of undefined

10-07-2019 04:39
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

10-07-2019 04:39
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
var dayname = new Array();
day[0] = "Mon";
day[1] = "Tue";
day[2] = "Wed";
day[3] = "Thu";
day[4] = "Fri";
day[5] = "Sat";
day[6] = "Sun";
let dayname = day[today.getDay]
You are declaring "dayname" as an array then using "day" as your array reference.
It should be:-
var day = new Array();
10-12-2019 04:59
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
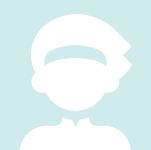
10-12-2019 04:59
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I have now updated this and it has changed the element which is meant to be the date to the day of the week and the day of the week is still displaying the same.

10-14-2019 02:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

10-14-2019 02:25
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Have you allowed enough space to display it properly in your text element?

10-14-2019 19:21
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
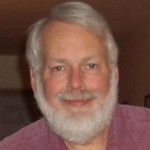
10-14-2019 19:21
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
The fact that the display shows undefined strongly suggests a typo.
Can you post the entire code from all files?
John

11-02-2019 08:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
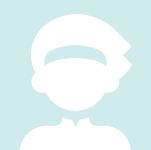
11-02-2019 08:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
index.js
import clock from "clock";
import document from "document";
import { preferences } from "user-settings";
import { zeroPad, } from "../common/utils";
import userActivity from "user-activity";
import * as simpleSettings from "./simple/device-settings.js";
// required imports
import * as kpay from './kpay/release/kpay.js';
import * as kpay_common from '../common/kpay/kpay_common.js';
import './kpay/release/kpay_filetransfer.js';
import './kpay/release/kpay_dialogs.js'; // remove if you handle KPay dialogs yourself
import './kpay/release/kpay_time_trial.js'; // remove if you do not want a time based trial
import './kpay/release/kpay_msg_validation.js';
/**** KPAY INIT - REQUIRED ***/
kpay.initialize();
// Update the clock every minute
clock.granularity = "minutes";
// Get a handle on the <text> elements specified in the index.gui file
const timeHandle = document.getElementById("timeLabel");
const stepsHandle = document.getElementById("stepsLabel");
const dayHandle = document.getElementById ("dayLabel");
const monthHandle = document.getElementById ("monthLabel");
const daynameHandle = document.getElementById ("daynameLabel");
// Update the <text> element every tick with the current time
clock.ontick = (evt) => {
let today = new Date();
let monthnum = today.getMonth();
let dayname = today.getDay();
let day = today.getDate();
var month = new Array();
month[0] = "Jan";
month[1] = "Feb";
month[2] = "Mar";
month[3] = "Apr";
month[4] = "May";
month[5] = "Jun";
month[6] = "Jul";
month[7] = "Aug";
month[8] = "Sep";
month[9] = "Oct";
month[10] = "Nov";
month[11] = "Dec";
let monthname = month[monthnum];
var day = new Array();
day[0] = "Mon";
day[1] = "Tue";
day[2] = "Wed";
day[3] = "Thu";
day[4] = "Fri";
day[5] = "Sat";
day[6] = "Sun";
let dayname = day[today.getDay]
const now = evt.date;
let hours = now.getHours();
let mins = now.getMinutes();
if (preferences.clockDisplay === "12h") {
hours = hours % 12 || 12;
} else {
hours = zeroPad(hours);
}
let minsZeroed = zeroPad(mins);
timeHandle.text = `${hours}:${minsZeroed}`;
monthHandle.text = `${monthname}`;
dayHandle.text = `${day}`;
daynameHandle.text = `${dayname}`;
// Activity Values: adjusted type
let stepsValue = (userActivity.today.adjusted["steps"] || 0);
let stepsString = stepsValue + ' steps';
stepsHandle.text = stepsString;
}
/* -------- SETTINGS -------- */
let stepsLabel = document.getElementById("stepsLabel");
let timeLabel = document.getElementById("timeLabel");
let dayLabel = document.getElementById("dayLabel");
let monthLabel = document.getElementById("monthLabel");
let dividerTop = document.getElementById ("divider-top");
let dividerBtm = document.getElementById ("divider-btm");
let daynameLabel = document.getElementById ("daynameLabel");
function settingsCallback(data){
if (!data){
return;
}
if (data.colorSteps) {
stepsLabel.style.fill = data.colorSteps;
}
if (data.colorTime) {
timeLabel.style.fill = data.colorTime;
}
if (data.colorDayname) {
daynameLabel.style.fill = data.colorDayname;
}
if (data.colorDay) {
dayLabel.style.fill = data.colorDay;
}
if (data.colorMonth) {
monthLabel.style.fill = data.colorMonth;
}
if (data.colorDivider) {
dividerTop.style.fill = data.colorDivider;
dividerBtm.style.fill = data.colorDivider;
}
}
simpleSettings.initialize(settingsCallback);
index.gui
<svg class="background">
<text id="timeLabel" />
<text id="stepsLabel" />
<text id="daynameLabel"/>
<text id="dayLabel" />
<text id="monthLabel"/>
<rect id="divider-top" />
<rect id="divider-btm" />
<!-- USE KPAY UI CODE - ADD ALL OWN UI CODE ABOVE THIS LINE -->
<use href="#kpay" />
</svg>
index.jsx
const colorSet = [
{color: "white"},
{color: "mistyrose"},
{color: "lightpink"},
{color: "crimson"},
{color: "#F83C40"},
{color: "red"},
{color: "orangered"},
{color: "orange"},
{color: "#FFCC33"},
{color: "yellow"},
{color: "#E4FA3C"},
{color: "#B8FC68"},
{color: "lightgreen"},
{color: "yellowgreen"},
{color: "olivedrab"},
{color: "seagreen"},
{color: "teal"},
{color: "lightskyblue"},
{color: "deepskyblue"},
{color: "dodgerblue"},
{color: "navy"},
{color: "indigo"},
{color: "darkviolet"},
{color: "#BD4EFC"},
{color: "grey"},
{color: "silver"},
{color: "lightgrey"}
];
const options = [
['Time Color', 'colorTime'],
['Divider Color', 'colorDivider'],
['Steps Color', 'colorSteps'],
['Day Color', 'colorDay'],
['Month Color', 'colorMonth']
['Dayname Color', 'colorDayname']
];
function mySettings(props) {
return (
<Page>
{options.map(([title, settingsKey]) =>
<Section
title={title}>
<ColorSelect
settingsKey={settingsKey}
colors={colorSet} />
</Section>
)}
</Page>
);
}
registerSettingsPage(mySettings);
style.css
.background {
viewport-fill: black;
}
#timeLabel {
font-size: 170;
font-family: Tungsten-Medium;
text-length: 5;
text-anchor: middle;
letter-spacing: 15;
x: 50%;
y: 70%;
fill: white;
}
#stepsLabel {
font-size: 45;
font-family: Fabrikat-Black;
text-length: 10;
text-anchor: middle;
letter-spacing: 3;
x: 50%;
y: 93%;
fill: white;
}
#monthLabel {
font-size: 50;
font-family: Fabrikat-Black;
text-length: 10;
text-anchor: middle;
letter-spacing: 4;
x: 63%;
y: 17%;
fill: white;
}
#daynameLabel{
font-size: 25;
font-family: Fabrikat-Black;
text-length: 300;
text-anchor: start;
letter-spacing: 5;
x: 15%;
y: 17%;
fill: white;
}
#dayLabel {
font-size: 50;
font-family: Fabrikat-Black;
text-length: 2;
text-anchor: middle;
letter-spacing: 5;
x: 40%;
y: 17%;
fill: white;
}
#divider-top {
x: 2%;
y: 24%;
width: 286;
height: 5;
fill: teal;
}
#divider-btm {
x: 2%;
y: 75%;
width: 286;
height: 5;
fill: teal;
}

11-02-2019 12:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
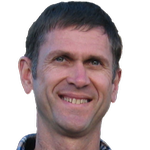
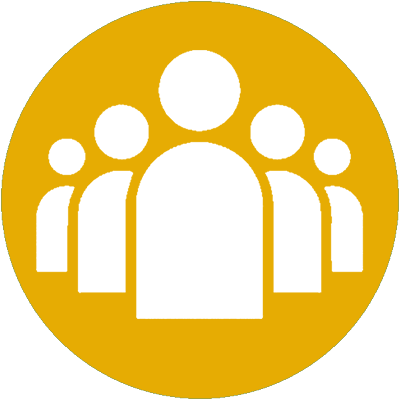
11-02-2019 12:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
getDay is a function: try getDay()
Gondwana Software

11-06-2019 16:48
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
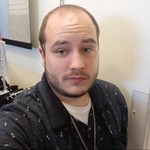
11-06-2019 16:48
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
...
let dayname = today.getDay();
...
var dayname = new Array();
...
let dayname = day[today.getDay]
...
So the problem I see is that you are using the same variable name in multiple places, overwriting what it was set to before.
Fix this and It should work.
Just a tip as well, put your arrays above 'clock.ontick' so that the object won't be created every time the clock tics (this will help with memory and probably save battery life as well

11-09-2019 04:22
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
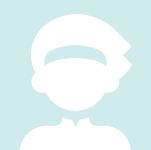
11-09-2019 04:22
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
When I run the watch face it still will not display the day of the week but the display on longer has undefined on it.
It still get two errors TypeError and Uncaught TypeError.

11-09-2019 07:50
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
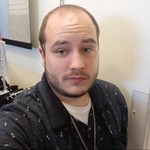
11-09-2019 07:50
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Just noticed one more error you maybe didn't notice yet:
You have "today.getDay" instead of "today.getDay()"
Forgot the "()"

