05-27-2018 02:30
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
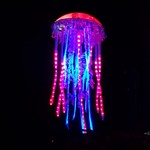
05-27-2018 02:30
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Hi There,
I am trying to reliably detect keys pressed when the screen is off. I can detect reliably when the screen is on but once the screen is off and a few seconds have passed I am finding some key presses are missed. repeated key presses improves reliability but often the first couple are missed. Is there a way to reliably detect key presses when the screen is off?
The simulator seems to stop detecting any keys once the screen has been turned off.
Thanks,
Brett.
Here is the code I'm using to test the problem:
import clock from "clock";
import document from "document";
import { preferences } from "user-settings";
import * as util from "../common/utils";
import { display } from "display";
// initialise global variables
var previousKey = ""
var previousKey2 = ""
var dateObj = new Date();
var previousKeyDate = dateObj.getTime()
var blankEnabled = false;
// Update the clock every minute
clock.granularity = "minutes";
// Get a handle on the <text> element
const myLabel = document.getElementById("myLabel");
// Update the <text> element every tick with the current time
clock.ontick = (evt) => {
let today = evt.date;
let hours = today.getHours();
if (preferences.clockDisplay === "12h") {
// 12h format
hours = hours % 12 || 12;
} else {
// 24h format
hours = util.zeroPad(hours);
}
let mins = util.zeroPad(today.getMinutes());
myLabel.text = `${hours}:${mins}`;
}
// Toggle Blank screen if key is pressed 3 times
document.onkeypress = function(e) {
var currentDate = new Date()
var dateInterval = currentDate.getTime() - previousKeyDate;
var maxInterval = 1500
console.log("Key pressed: " + e.key + " Previous Key pressed: " + previousKey + " Previous Previous Key: " + previousKey2);
console.log("current date: " + currentDate.getTime() + " previous key date: " + previousKeyDate + " Date Interval: " + (currentDate.getTime() - previousKeyDate) );
if (blankEnabled == false & e.key == previousKey & previousKey != "reset" & e.key == previousKey2 & dateInterval < maxInterval ) {
previousKey = "reset"
previousKey2 = "reset"
blankEnabled = true;
display.on = false
console.log("Blank Screen Enabled");
} else if (e.key == previousKey & previousKey != "reset" & e.key == previousKey2 & dateInterval < maxInterval) {
previousKey = "reset"
previousKey2 = "reset"
blankEnabled = false;
display.on = true;
console.log("Blank Screen Disabled");
} else {
previousKey2 = previousKey
previousKey = e.key
console.log("Previous Key Updated")
}
previousKeyDate = currentDate.getTime();
}
//detect Screen changing state and turn off again
display.onchange = function() {
console.log("Screen state change detected");
if (blankEnabled) {
console.log("Screen turned off");
display.on = false
}
}

