11-21-2017 13:41
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
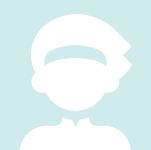
Jogger
1
0
0
11-21-2017 13:41
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I'm working on a Java app where I use the Fitbit API to pull the user's information. But when I try to pull information about the user I get the following error:
org.springframework.web.client.HttpClientErrorException: 400 Bad Request org.springframework.web.client.DefaultResponseErrorHandler.handleError(DefaultResponseErrorHandler.java:91) com.cc.ehealth.fitbit.web.WebTransport$CustomResponseErrorHandler.handleError(WebTransport.java:59) org.springframework.web.client.RestTemplate.handleResponse(RestTemplate.java:641) org.springframework.web.client.RestTemplate.doExecute(RestTemplate.java:597) org.springframework.web.client.RestTemplate.execute(RestTemplate.java:557) org.springframework.web.client.RestTemplate.exchange(RestTemplate.java:475) com.cc.ehealth.fitbit.web.WebTransport.postForm(WebTransport.java:38) com.cc.ehealth.fitbit.oauth.OauthService.refreshToken(OauthService.java:73) com.cc.ehealth.fitbit.resource.ResourceAspect.refreshAccessTokenIfNeeded(ResourceAspect.java:36) sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) java.lang.reflect.Method.invoke(Method.java:498) org.springframework.aop.aspectj.AbstractAspectJAdvice.invokeAdviceMethodWithGivenArgs(AbstractAspectJAdvice.java:621) org.springframework.aop.aspectj.AbstractAspectJAdvice.invokeAdviceMethod(AbstractAspectJAdvice.java:610) org.springframework.aop.aspectj.AspectJAroundAdvice.invoke(AspectJAroundAdvice.java:68) org.springframework.aop.framework.ReflectiveMethodInvocation.proceed(ReflectiveMethodInvocation.java:179) org.springframework.aop.interceptor.ExposeInvocationInterceptor.invoke(ExposeInvocationInterceptor.java:92) org.springframework.aop.framework.ReflectiveMethodInvocation.proceed(ReflectiveMethodInvocation.java:179) org.springframework.aop.framework.JdkDynamicAopProxy.invoke(JdkDynamicAopProxy.java:207) com.sun.proxy.$Proxy65.getUserInfo(Unknown Source) com.cc.chealth.services.HealthSyncServiceImpl.validateFitbitToken(HealthSyncServiceImpl.java:237) com.cc.chealth.PortalController.goToHealthJSP(PortalController.java:270) sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) java.lang.reflect.Method.invoke(Method.java:498) org.springframework.web.method.support.InvocableHandlerMethod.doInvoke(InvocableHandlerMethod.java:221) org.springframework.web.method.support.InvocableHandlerMethod.invokeForRequest(InvocableHandlerMethod.java:137) org.springframework.web.servlet.mvc.method.annotation.ServletInvocableHandlerMethod.invokeAndHandle(ServletInvocableHandlerMethod.java:110) org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.invokeHandlerMethod(RequestMappingHandlerAdapter.java:806) org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter.handleInternal(RequestMappingHandlerAdapter.java:729) org.springframework.web.servlet.mvc.method.AbstractHandlerMethodAdapter.handle(AbstractHandlerMethodAdapter.java:85) org.springframework.web.servlet.DispatcherServlet.doDispatch(DispatcherServlet.java:959) org.springframework.web.servlet.DispatcherServlet.doService(DispatcherServlet.java:893) org.springframework.web.servlet.FrameworkServlet.processRequest(FrameworkServlet.java:970) org.springframework.web.servlet.FrameworkServlet.doGet(FrameworkServlet.java:861) javax.servlet.http.HttpServlet.service(HttpServlet.java:622) org.springframework.web.servlet.FrameworkServlet.service(FrameworkServlet.java:846) javax.servlet.http.HttpServlet.service(HttpServlet.java:729) org.apache.tomcat.websocket.server.WsFilter.doFilter(WsFilter.java:52) com.opensymphony.sitemesh.webapp.SiteMeshFilter.obtainContent(SiteMeshFilter.java:129) com.opensymphony.sitemesh.webapp.SiteMeshFilter.doFilter(SiteMeshFilter.java:77)
I have narrowed down the error to the following functions:
(the app gives the error at the validateFitbitToken() function)
@RequestMapping(value = { "/health" }, method = RequestMethod.GET) public String goToHealthJSP(HttpServletRequest request) { Object u = request.getSession().getAttribute("account"); if (u == null) { return "redirect:/"; } else { //commented out for now //request.getSession().removeAttribute("errorMsg"); // Check if the user is logged into fitbit by seeing if they have the fitbit-auth flag set. System.out.println("portalcontroller before users----- "); User user = (User) u; System.out.println("this is users " + user); System.out.println("here 1"); UserInfo fitbitUserInfo = healthSyncService.validateFitbitToken(user); System.out.println("here 2"); request.setAttribute("fitbitAuth", user.isFitbitAuth()); System.out.println("here 3"); if (user.isFitbitAuth()) { // Refresh the user's health data from the Fitbit API, required for all page loads and reloads System.out.println("here 4"); System.out.println("this is the user: " + user); //System.out.println("/n this is the user's fitbit info: " + fitbitUserInfo); healthSyncService.refreshSync(user, fitbitUserInfo); System.out.println("here 5"); } System.out.println("portalcontroller after users----- "); return "portal/health"; } }
This is the ValidateFitbitToken function, and it stop working at the getUserInfo() function
@Override public UserInfo validateFitbitToken(User user) { System.out.println("inside validateFitbitTokeb"); if (!user.isFitbitAuth()) { // Short circuit method. No token, so no Fitbit link. System.out.println("no token"); return null; } System.out.println("yes token"); OauthAccessToken fitbitToken = user.getFitbitAuthToken(); System.out.println("token set"); System.out.println(fitbitToken); //Works till here UserInfo userInfo = resourceService.getUserInfo(fitbitToken); System.out.println("user info got"); System.out.print("this is user info" + userInfo); if (userInfo == null && !user.isFitbitAuth()) { // The fitbit access token was invalid, so we will need to System.out.println("access token invalid"); user.fitbitOauth2AccessToken = null; user.fitbitOauth2RefreshToken = null; mergeService.saveFitbitToken(user); } System.out.println("About to print user info"); return userInfo; }
I believe that the token I set is working but I'm not really sure what is causing the issue.
Any help is appreciated! I can provide more information or code if necessary.
Thanks!
0 REPLIES 0
