11-17-2020 13:35
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
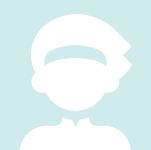
11-17-2020 13:35
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
For simplicity, I'm going to pare down my app to the very essence of this problem. I will provide here all of the relevant code.
Summary:
When you tap something in the app, it creates a new thing and adds it to an array of things.
Each Thing object has a "name" property. The a corresponding list of <TextInput> components should appear in the Settings, and update whenever a Thing is added. When the user updates the input text, it should update the corresponding Thing's attribute in the app.
Here is my very simple code:
Thing.js:
export default class Thing {
constructor() {
this.name = "default";
this.message = "Hello!";
}
sayHi() {
console.log(this.message);
}
}
index.js:
import document from "document";
import Thing from "./Thing";
let bg = document.getElementById("bg");
let things = [];
let i = 0;
bg.addEventListener("click", evt => {
things[i++] = new Thing();
console.log(`Thing added. There are now ${i} things.`);
});
Any help would be very much appreciated. I think I simply lack an adequate understanding of JSX and the JavaScript required, but i hope someone can help me learn here.
11-17-2020 14:17
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
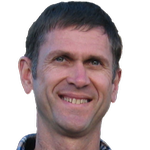
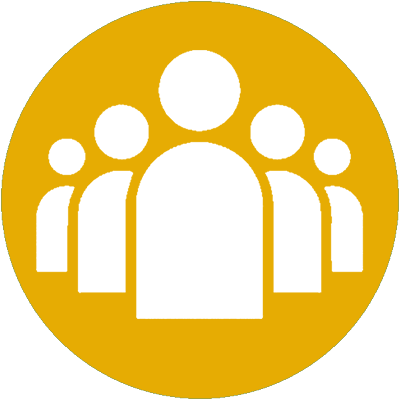
11-17-2020 14:17
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I may be misunderstanding, but...
In general terms, I think you'll need to communicate from watch to companion whenever a new Thing is created. The companion will need to update a settingsStorage (setting) entry to accommodate the new Thing. Your .jsx will need to react to changes to that setting, which should be automatic if you use {} correctly.
I'm not sure you can dynamically create <TextInput> entries in .jsx, but I've never tried. I've created some apps where the array of Things is shown in a .jsx <Select>. When selected, the corresponding Thing can be edited using a <TextInput> (ie, there's only one <TextInput> which is used for all Things).
I'd recommend getting the companion and .jsx side of this working, before trying to connect it to the watch. You might find that the limitations of settings prompts you to do things a bit differently.
In my case, I also create the Things in .jsx code. The watch gets informed when the Thing has been created and initialised.
You might find that your companion and settings become 'stateful', which is a problem when components can be stopped at any time, communications can be slow or lost, and users may not see things through to completion. Try to protect yourself from those eventualities.
Gondwana Software

