07-21-2018 23:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
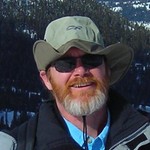
07-21-2018 23:14
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I am working with the basic clock app tutorial and have modified it to be able to switch background images. In the main program I have a variable that does change value. However in the resources section and in the index.gui I have the following...
<svg class="background">
<image x="0" y="0" href = imgFile load="sync" />
<text id="myLabel"></text>
<text id="myLabel1"></text>
</svg>
the "imgFile" is the new file name I want the program to pull the image. The main program in the apps section in index.js the code that changes the imgFile variable looks like
if (oldhour != secs) {
imgcnt = imgcnt +1;
oldhour = secs;
if (imgcnt > maximg) imgcnt=1;
imgFile = imgcnt + '.jpg';
console.log (imgFile)
}
Yes, this does show in the console that the imgFile changes every second. But I get an error in the .gui of Error 22 Bad SVGML 'imgFile load="sync" /'
What am I doing wrong? I am new to programming in Java.

07-22-2018 00:54
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
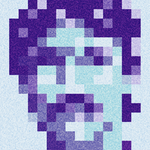
07-22-2018 00:54
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
It looks like you are passing the variable imgFile directly into the SVG
<image x="0" y="0" href=imgFile load="sync" />
Which unfortunately won't work because at present it's not possible to pass variables into SVG like this. There are different ways to solve what you would like to do. One of the ways I can think with the effect closest to your intended code would be to give the image element an id and a starting image value:
<image id="img" href="0.jpg" load="sync" />
and then access the href property of the element from Javascript
const img = document.getElementById("img") if (timeForImgChange) {
// change imgCnt value somewhere...
img.href = imgCnt + ".jpg"; }
Another way, if there are not too many images, would be to add them all to the SVG code as image elements with unique id's and hide/show them from Javascript. This would allow more flexibility for adding transitions or animations if wanted.

07-22-2018 08:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
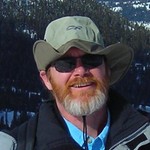
07-22-2018 08:40
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
@sbtnNice information, but I am not savvy as to where to put what code where. I tried, but no luck. Do you have an example of what I put in the index.gui and what goes in the index.js?
I would be interested to see your other option in code as well. Though animations are not necessary.
Ideally it would be nice to be able to push a couple of different files and then access them so that a user can change the images at their leisure. But I then think how would one change the files and where are they stored.

07-22-2018 12:40 - edited 07-22-2018 12:53
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
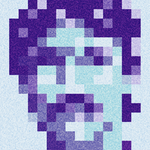
07-22-2018 12:40 - edited 07-22-2018 12:53
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Sure, for the solution changing the href attribute, the relevant code would be in index.gui and index.js (plus your images 0.jpg and 1.jpg being in the resources folder).
index.gui:
<svg> <image id="img" href="0.jpg" load="sync" /> </svg>
index.js:
import document from "document";
import clock from "clock";
let img = document.getElementById("img");
let imageIndex = 0;
function changeImage() {
img.href = imageIndex + ".jpg";
console.log("image: " + img.href);
imageIndex += 1;
if (imageIndex > 1) {
imageIndex = 0;
}
};
clock.granularity = "seconds";
clock.ontick = function() {
changeImage();
};
I have used the clock tick every second to keep it simple, but it can be done with any method of counter.
Another approach would be to hard-code the images first into the SVG and use the animation options there, which you later trigger from Javascipt. Right now there is no way to dynamically create new SVG elements, which might be good to know as you consider your options. The SVG file would then look like this:
index.gui:
<svg> <image id="img1" href="0.jpg" load="sync"> <animate attributeName="opacity" begin="enable" from="0" to="1" dur="0.5" /> <animate attributeName="opacity" begin="disable" from="1" to="0" dur="0.5" /> </image> <image id="img2" href="1.jpg" load="sync"> <animate attributeName="opacity" begin="enable" from="0" to="1" dur="0.5" /> <animate attributeName="opacity" begin="disable" from="1" to="0" dur="0.5" /> </image> </svg>
index.js:
import document from "document"; import clock from "clock"; const img1 = document.getElementById("img1"); const img2 = document.getElementById("img2"); const images = [img1, img2]; let imageIndex = 0; function changeImage() { images[imageIndex].animate("enable"); imageIndex += 1; if (imageIndex > 1) { imageIndex = 0; } images[imageIndex].animate("disable"); }; clock.granularity = "seconds"; clock.ontick = function() { changeImage(); };
The actual difference in the 2 methods above is shown in these 2 gifs, the first one is changing out the href attribute and the second with the SVG animation.
I haven't really explored it but I think the Image picker/Image API might be what you are looking for to solve your idea with user added images. I'm sure there are others with more experience in that who can help you out!

07-22-2018 20:26
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
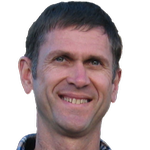
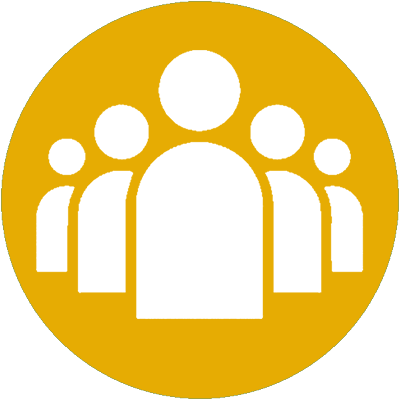
07-22-2018 20:26
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Does this seem like the sort of thing you're trying to do?
Gondwana Software

07-27-2018 11:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
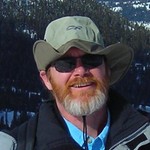
07-27-2018 11:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Derek

