09-04-2015 21:28 - edited 09-04-2015 22:11
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
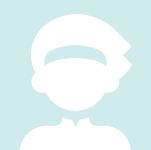
09-04-2015 21:28 - edited 09-04-2015 22:11
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I've put together a sample .Net program in C# and VB.Net.
It enables you to output JSON format data as CSV file data, for use in programs like Excel, such as this 4 day chart:
Here's what it does:
- The program takes JSON replies from a file called JSON_Data.txt that will be in your EXE folder (/bin/debug or /bin/release), filtering out empty lines.
- Deserialises them into a class structure.
- Skims off the Heart-Rate data, and concatonates all the JSON lines into a single List<DataPoint>
- Orders the List<DataPont> by sample date.
- Creates a .CSV file, containing the List<DataPoint> data.
The file can be loaded into any program that supports CSV files.
I hope you find the sample code useful!
IMPORTANT!
This program uses .Net 4.0, and NOT 4,0 Client profile, because you need to add in the references "system.web.extensions.dll", which is not available in the Client-profile platform.
To run the code - create a new .Net "Console Application", and copy and paste the code into the main .CS, or .VB file that is reated. Hit F5 to compile and run it. (make sure you've got some JSON data in that text file!)
VB.Net 4.0
Imports System.Web.Script.Serialization ' Sytem.web.extensions.dll Imports System.IO Imports System.Text Module Module1 public serializer As New JavaScriptSerializer() Sub Main() Dim fileData() As String = File.ReadAllText("JSON_Data.txt").Split(vbLf) Dim datapoints As New List(Of DataPoint) For Each jsonData As String In fileData If jsonData.Trim() <> "" Then datapoints.AddRange(getDeserialisedChargeHRData(jsonData)) Next Dim sortedDataPoints As List(Of DataPoint) = datapoints.OrderBy(Function(o) o.dateTime).ToList() Dim sb As New StringBuilder() sb.AppendLine("Date and time,BPM,Confidence,Calories burned,Default zone,Custom zone") For Each dp As DataPoint In datapoints sb.AppendLine(String.Format("{0},{1},{2},{3},""{4}"",""{5}""", dp.dateTime, dp.bpm, dp.confidence, dp.caloriesBurned, dp.defaultZone, dp.customZone)) Next File.WriteAllText("BMP_Data.csv", sb.ToString()) End Sub Public Function getDeserialisedChargeHRData(ByVal json As String) As List(Of DataPoint) Dim deserializedResult = serializer.Deserialize(Of List(Of RootObject))(json) Return deserializedResult.Item(0).dataSets.activity.dataPoints End Function End Module Public Class DataPoint Public Property bpm() As Integer Public Property confidence() As Integer Public Property caloriesBurned() As Double Public Property defaultZone() As String Public Property customZone() As String Public Property dateTime() As Date End Class Public Class Activity Public Property dataPoints() As List(Of DataPoint) Public Property granularity() As String End Class Public Class DataSets Public Property activity() As Activity End Class Public Class RootObject Public Property dataSets() As DataSets End Class
C# .Net 4.0
using System; using System.Collections; using System.Collections.Generic; using System.Data; using System.Diagnostics; using System.Web.Script.Serialization; // Sytem.web.extensions.dll using System.IO; using System.Text; static class Module1 { public static JavaScriptSerializer serializer = new JavaScriptSerializer(); public static void Main() { string[] fileData = File.ReadAllText("JSON_Data.txt").Split("\r"); List<DataPoint> datapoints = new List<DataPoint>(); foreach (string jsonData in fileData) { if (!string.IsNullOrEmpty(jsonData.Trim())) datapoints.AddRange(getDeserialisedChargeHRData(jsonData)); } List<DataPoint> sortedDataPoints = datapoints.OrderBy(o => o.dateTime).ToList(); StringBuilder sb = new StringBuilder(); sb.AppendLine("Date and time,BPM,Confidence,Calories burned,Default zone,Custom zone"); foreach (DataPoint dp in datapoints) { sb.AppendLine(string.Format("{0},{1},{2},{3},\"{4}\",\"{5}\"", dp.dateTime, dp.bpm, dp.confidence, dp.caloriesBurned, dp.defaultZone, dp.customZone)); }
File.WriteAllText("BMP_Data.csv", sb.ToString()); } public static List<DataPoint> getDeserialisedChargeHRData(string json) { dynamic deserializedResult = serializer.Deserialize<List<RootObject>>(json); return deserializedResult.Item(0).dataSets.activity.dataPoints; } } public class DataPoint { public int bpm { get; set; } public int confidence { get; set; } public double caloriesBurned { get; set; } public string defaultZone { get; set; } public string customZone { get; set; } public System.DateTime dateTime { get; set; } } public class Activity { public List<DataPoint> dataPoints { get; set; } public string granularity { get; set; } } public class DataSets { public Activity activity { get; set; } } public class RootObject { public DataSets dataSets { get; set; } }
09-21-2015 23:00
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

09-21-2015 23:00
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Nice one! Out of interest, was there any reason in particular why you didnt do it using a PowerShell script? I'm not being a smartass... I usually prefer visual studio too cause of the debugging tool but I already have PShell scripts for Rest API functions, and am likely to be making some Web API calls too.

09-22-2015 01:47
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
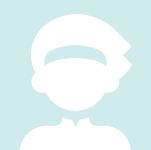
09-22-2015 01:47
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
using a PowerShell script? I'm not being a smartass... I usually prefer
visual studio too cause of the debugging tool
I've never used PowerShell!
I've done lots with COM objects and VBScript in the past (good old
script.exe)
I wrote it in VS so people could use it in .Net websites, and Winform/WPF
apps just by copying and pasting it in.
I'm very weak converting JSON back to class structures - so I used this as
practice, and double checked with some online conversion tools.
What kinds of things are you trying with the data?

11-03-2016 18:38 - edited 11-03-2016 18:41
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

SunsetRunner
11-03-2016 18:38 - edited 11-03-2016 18:41
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Hi,
I tired to use the same code to generate .csv file from json. I was getting the following error.
System.ArgumentOutOfRangeException:
Index was out of range. Must be non-negative and less than the size of the collection.
at Main.getDeserialisedChargeHRData(String) in Main.vb:line 44
at Main.Main() in Main.vb:line 23
It will be useful, if you can provide me the sample json file("JSON_Data.txt") used to execute the code.

11-03-2016 22:38
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
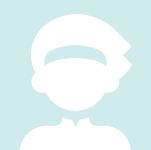
11-03-2016 22:38
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Hi Raj,
Here's the data I used - multiple JSON replies from the website pasted into a text document:
11-03-2016 23:55
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post

SunsetRunner
11-03-2016 23:55
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Thanks, it worked. Just wanted to know, is there any generic code to generate CSV file for Json data text file (I mean irrespective of data structure in JSON file). Just like in few websites, they are providing csv or excel files irrespective of structure of data in json file.

