07-08-2019 00:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
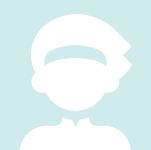
07-08-2019 00:49
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Hello,
I am working on an app that records accelerometer,gyroscope data, and gps data. It stores this as binary into a .bin file. That .bin is transferred to the companion (android phone) where I intend to use a fetch command to POST it to a http server on the phone to easily get the file.
Problems I am running into:
I create the .bin file and data is being written to it (I read data back some of the data in the file to ensure its working). When I run this code:
//QUEU uP FOR TRANSFER outbox.enqueueFile(filename).then((ft) => { console.log(`Transfer of ${ft.name} successfully queued.`); }) .catch((error) => { console.log(`Failed to schedule transfer: ${error}`); })
This returns successfully queued.
On the companion side I run this code:
inbox.addEventListener("newfile", processAllFiles); async function processAllFiles() { let file; while ((file = await inbox.pop())) { const newbuffer = await file.text(); console.log(`file contents:`,newbuffer); } } processAllFiles();
The data returned is always null.
Additionally I am trying to run an HTTP POST using this code also on the companion:
var formData = new FormData(); var fileField = document.querySelector('.bin'); formData.append('test', fileField.files[0]); fetch('http://*insertIPAddressHere*', { method: 'PUT', body: formData }) .then(response => response.json()) .catch(error => console.error('Error:', error)) .then(response => console.log('Success:', JSON.stringify(response)));
This is the first time writing Javascript. Am I on the right track?

07-08-2019 00:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
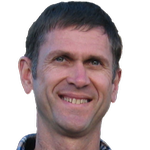
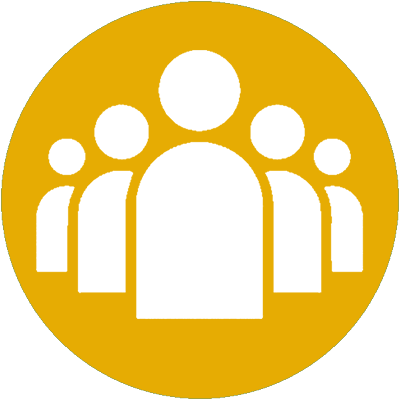
07-08-2019 00:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Just a couple of quick and very possibly wrong observations:
- file.text() may not be what you want if the data is binary
- Fitbit's implementation of fetch() may only work with https.
Gondwana Software
07-08-2019 01:02 - edited 07-08-2019 01:03
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
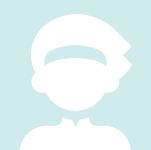
07-08-2019 01:02 - edited 07-08-2019 01:03
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
I thought that as well.
I attempted this code for the binary but that also didn't work:
async function processAllFiles() { let file; while ((file = await inbox.pop())) { let newbuffer = new Float32Array(8); newbuffer = await file.arrayBuffer(); console.log(`file contents:`,newbuffer); } } processAllFiles();
Does HTTP not work for a local network address? It says 'except when accessing resources on a local network by IP address.'

07-08-2019 01:44
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
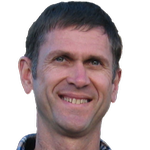
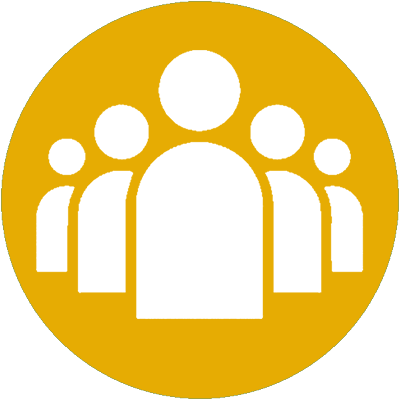
07-08-2019 01:44
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
I can't see why arrayBuffer() wouldn't work. It might be informative to take a look at file.length beforehand, to see if it's sensible.
And I think you're right about http.
Gondwana Software
07-08-2019 02:15
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
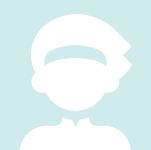
07-08-2019 02:15
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
the file is the correct size as to what I am sending.
3200 bytes do arrive.
async function processAllFiles() { let file; while ((file = await inbox.pop())) { console.log(file.length); let newbuffer = new Float32Array(100); newbuffer = await file.arrayBuffer(); console.log(`file contents:`,newbuffer); } } processAllFiles();
I can't seem to get it to read correctly. I thought maybe the buffer was too small to hold the entire file so I increased the size to match the fileLength but still nothing appears. newbuffer.length is undefined.

07-08-2019 02:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
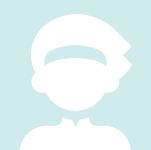
07-08-2019 02:57
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
- Who Voted for this post?
Solved!
async function processAllFiles() { let file; while ((file = await inbox.pop())) { const newbuffer = await file.arrayBuffer(); let newbuffer2 = new Float32Array(newbuffer) } }
newbuffer2 is now an array which you can increment through as any other array.
07-08-2019 06:48
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
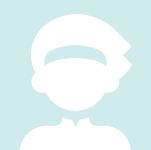
07-08-2019 06:48
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
What is the best Method to get the logging data from the companion after it was transferred from the device?
Is it expected we write our own android webserver that can handle fetch commands?

07-08-2019 14:23
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
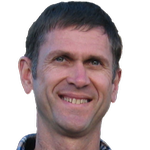
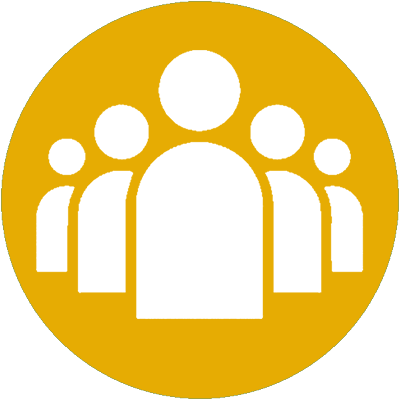
07-08-2019 14:23
- Mark as New
- Bookmark
- Subscribe
- Permalink
- Report this post
Great! I did wonder whether
Float32Array(100)
might have been too small for 3200 bytes, but that may not have caused the problem you observed.
Gondwana Software

